How To Get An Element From A Set In Python
A gear up is an unordered collection of items. Every gear up element is unique (no duplicates) and must be immutable (cannot be changed).
However, a set itself is mutable. Nosotros can add together or remove items from information technology.
Sets can also be used to perform mathematical set operations like matrimony, intersection, symmetric difference, etc.
Creating Python Sets
A prepare is created by placing all the items (elements) inside curly braces {}
, separated by comma, or past using the born set()
function.
Information technology tin take whatever number of items and they may be of different types (integer, float, tuple, string etc.). But a set cannot have mutable elements like lists, sets or dictionaries as its elements.
# Unlike types of sets in Python # set of integers my_set = {1, 2, 3} print(my_set) # set up of mixed datatypes my_set = {i.0, "Hello", (1, 2, 3)} impress(my_set)
Output
{1, 2, 3} {1.0, (1, ii, 3), 'Hello'}
Try the following examples besides.
# set cannot accept duplicates # Output: {one, two, 3, 4} my_set = {1, 2, three, 4, three, 2} print(my_set) # we can make set from a list # Output: {1, 2, 3} my_set = set up([1, 2, 3, ii]) impress(my_set) # gear up cannot have mutable items # here [three, 4] is a mutable listing # this volition cause an error. my_set = {one, 2, [3, four]}
Output
{1, two, 3, four} {ane, 2, 3} Traceback (most recent call final): File "<string>", line 15, in <module> my_set = {1, 2, [3, 4]} TypeError: unhashable type: 'listing'
Creating an empty set up is a chip tricky.
Empty curly braces {}
will make an empty dictionary in Python. To make a set without whatever elements, we use the prepare()
part without any argument.
# Distinguish gear up and dictionary while creating empty set # initialize a with {} a = {} # cheque data blazon of a print(blazon(a)) # initialize a with set() a = set() # check data type of a impress(type(a))
Output
<class 'dict'> <course 'set'>
Modifying a ready in Python
Sets are mutable. Yet, since they are unordered, indexing has no significant.
We cannot access or change an element of a prepare using indexing or slicing. Gear up data type does not support information technology.
Nosotros can add a unmarried element using the add()
method, and multiple elements using the update()
method. The update()
method can accept tuples, lists, strings or other sets as its statement. In all cases, duplicates are avoided.
# initialize my_set my_set = {1, 3} print(my_set) # my_set[0] # if you uncomment the above line # you will get an fault # TypeError: 'set up' object does not back up indexing # add an element # Output: {i, ii, 3} my_set.add(2) print(my_set) # add multiple elements # Output: {ane, ii, 3, 4} my_set.update([2, 3, 4]) print(my_set) # add list and set # Output: {1, ii, 3, 4, five, half-dozen, 8} my_set.update([four, 5], {one, vi, 8}) impress(my_set)
Output
{1, iii} {one, ii, iii} {ane, 2, 3, four} {1, 2, 3, four, five, 6, eight}
Removing elements from a ready
A particular item tin can be removed from a set using the methods discard()
and remove()
.
The simply deviation between the two is that the discard()
office leaves a set unchanged if the element is not present in the set. On the other paw, the remove()
function will heighten an fault in such a condition (if element is non present in the set).
The post-obit example will illustrate this.
# Difference between discard() and remove() # initialize my_set my_set = {1, iii, 4, 5, 6} print(my_set) # discard an element # Output: {one, 3, five, 6} my_set.discard(4) print(my_set) # remove an chemical element # Output: {1, iii, 5} my_set.remove(half dozen) print(my_set) # discard an element # not nowadays in my_set # Output: {1, iii, 5} my_set.discard(2) impress(my_set) # remove an element # not present in my_set # you volition get an error. # Output: KeyError my_set.remove(2)
Output
{1, iii, 4, five, half-dozen} {ane, 3, 5, 6} {1, 3, 5} {1, 3, v} Traceback (most recent call last): File "<string>", line 28, in <module> KeyError: two
Similarly, we can remove and render an detail using the popular()
method.
Since fix is an unordered data type, at that place is no way of determining which item will be popped. It is completely arbitrary.
We can also remove all the items from a prepare using the clear()
method.
# initialize my_set # Output: fix of unique elements my_set = fix("HelloWorld") print(my_set) # pop an element # Output: random element print(my_set.pop()) # pop another chemical element my_set.pop() impress(my_set) # articulate my_set # Output: fix() my_set.clear() print(my_set)
Output
{'H', 'l', 'r', 'Westward', 'o', 'd', 'e'} H {'r', 'W', 'o', 'd', 'e'} ready()
Python Set Operations
Sets tin can be used to deport out mathematical set operations like union, intersection, difference and symmetric difference. We can practice this with operators or methods.
Allow us consider the following 2 sets for the following operations.
>>> A = {1, ii, 3, 4, 5} >>> B = {iv, 5, 6, vii, 8}
Gear up Union
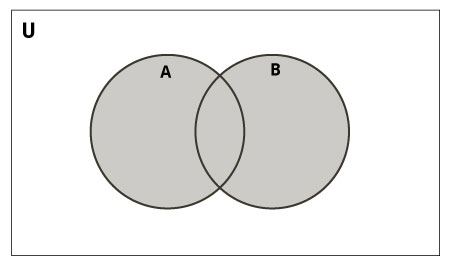
Marriage of A and B is a set of all elements from both sets.
Union is performed using |
operator. Aforementioned can be accomplished using the union()
method.
# Set wedlock method # initialize A and B A = {i, 2, iii, 4, 5} B = {4, 5, 6, 7, 8} # use | operator # Output: {i, two, iii, 4, 5, 6, 7, viii} print(A | B)
Output
{i, ii, iii, iv, 5, 6, vii, 8}
Try the following examples on Python shell.
# use matrimony function >>> A.union(B) {one, 2, 3, 4, 5, six, 7, 8} # use union function on B >>> B.spousal relationship(A) {1, 2, three, four, 5, 6, 7, eight}
Ready Intersection
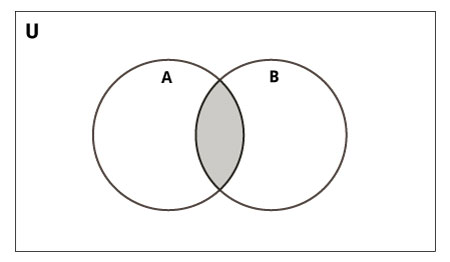
Intersection of A and B is a set of elements that are common in both the sets.
Intersection is performed using &
operator. Same can exist accomplished using the intersection()
method.
# Intersection of sets # initialize A and B A = {1, 2, 3, four, v} B = {4, 5, 6, 7, 8} # utilize & operator # Output: {4, 5} impress(A & B)
Output
{4, 5}
Endeavour the following examples on Python shell.
# utilize intersection function on A >>> A.intersection(B) {4, 5} # use intersection office on B >>> B.intersection(A) {4, 5}
Set Divergence
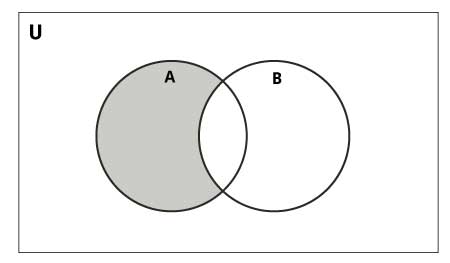
Difference of the prepare B from set A(A - B) is a fix of elements that are only in A but not in B. Similarly, B - A is a set of elements in B but not in A.
Difference is performed using -
operator. Same tin be achieved using the departure()
method.
# Divergence of two sets # initialize A and B A = {i, 2, 3, 4, v} B = {iv, 5, vi, vii, 8} # use - operator on A # Output: {1, 2, 3} print(A - B)
Output
{one, two, 3}
Try the following examples on Python shell.
# apply difference role on A >>> A.departure(B) {1, 2, 3} # use - operator on B >>> B - A {viii, 6, 7} # utilise departure function on B >>> B.difference(A) {viii, half dozen, 7}
Set Symmetric Divergence
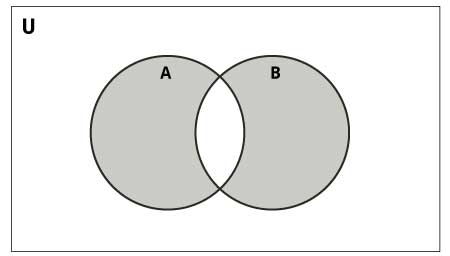
Symmetric Divergence of A and B is a set up of elements in A and B but non in both (excluding the intersection).
Symmetric difference is performed using ^
operator. Same can be accomplished using the method symmetric_difference()
.
# Symmetric difference of two sets # initialize A and B A = {i, two, iii, 4, 5} B = {iv, 5, six, 7, 8} # apply ^ operator # Output: {1, 2, 3, 6, 7, 8} impress(A ^ B)
Output
{1, ii, 3, 6, 7, viii}
Try the following examples on Python trounce.
# apply symmetric_difference role on A >>> A.symmetric_difference(B) {1, 2, three, 6, 7, 8} # utilize symmetric_difference part on B >>> B.symmetric_difference(A) {1, ii, 3, half-dozen, 7, 8}
Other Python Set Methods
In that location are many ready methods, some of which we have already used in a higher place. Here is a list of all the methods that are bachelor with the fix objects:
Method | Description |
---|---|
add() | Adds an element to the set up |
clear() | Removes all elements from the set |
copy() | Returns a copy of the set up |
departure() | Returns the divergence of two or more than sets every bit a new prepare |
difference_update() | Removes all elements of some other set from this set up |
discard() | Removes an element from the set if information technology is a member. (Exercise nothing if the chemical element is not in ready) |
intersection() | Returns the intersection of two sets equally a new fix |
intersection_update() | Updates the set up with the intersection of itself and another |
isdisjoint() | Returns Truthful if two sets accept a zero intersection |
issubset() | Returns True if another gear up contains this set |
issuperset() | Returns True if this fix contains another set |
pop() | Removes and returns an capricious set up element. Raises KeyError if the gear up is empty |
remove() | Removes an chemical element from the set. If the element is not a member, raises a KeyError |
symmetric_difference() | Returns the symmetric departure of 2 sets as a new set |
symmetric_difference_update() | Updates a ready with the symmetric difference of itself and another |
union() | Returns the union of sets in a new gear up |
update() | Updates the prepare with the wedlock of itself and others |
Other Fix Operations
Fix Membership Test
We can test if an item exists in a set or non, using the in
keyword.
# in keyword in a set # initialize my_set my_set = set("apple") # cheque if 'a' is present # Output: Truthful print('a' in my_set) # check if 'p' is present # Output: Fake print('p' non in my_set)
Output
True False
Iterating Through a Set up
Nosotros can iterate through each particular in a gear up using a for
loop.
>>> for letter of the alphabet in ready("apple tree"): ... print(alphabetic character) ... a p e fifty
Born Functions with Set
Built-in functions like all()
, whatever()
, enumerate()
, len()
, max()
, min()
, sorted()
, sum()
etc. are normally used with sets to perform different tasks.
Function | Description |
---|---|
all() | Returns Truthful if all elements of the prepare are true (or if the set is empty). |
any() | Returns True if any chemical element of the set is true. If the ready is empty, returns False . |
enumerate() | Returns an enumerate object. It contains the index and value for all the items of the fix as a pair. |
len() | Returns the length (the number of items) in the set. |
max() | Returns the largest item in the set. |
min() | Returns the smallest item in the fix. |
sorted() | Returns a new sorted list from elements in the set(does not sort the set itself). |
sum() | Returns the sum of all elements in the set. |
Python Frozenset
Frozenset is a new class that has the characteristics of a set, but its elements cannot be changed once assigned. While tuples are immutable lists, frozensets are immutable sets.
Sets being mutable are unhashable, so they can't be used as dictionary keys. On the other hand, frozensets are hashable and tin can exist used equally keys to a lexicon.
Frozensets can exist created using the frozenset() part.
This data type supports methods like copy()
, difference()
, intersection()
, isdisjoint()
, issubset()
, issuperset()
, symmetric_difference()
and union()
. Being immutable, information technology does non have methods that add or remove elements.
# Frozensets # initialize A and B A = frozenset([ane, ii, 3, 4]) B = frozenset([iii, four, 5, half-dozen])
Try these examples on Python shell.
>>> A.isdisjoint(B) False >>> A.difference(B) frozenset({1, 2}) >>> A | B frozenset({1, 2, 3, 4, v, 6}) >>> A.add(3) ... AttributeError: 'frozenset' object has no attribute 'add'
Source: https://www.programiz.com/python-programming/set
0 Response to "How To Get An Element From A Set In Python"
Post a Comment